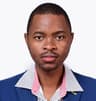
Tech Wizard
Published on β’ π3 min
How to Execute Something else after the Return Statement in Javascript
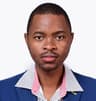
Tech Wizard
Published on β’ π6 min
Authentication in NextJS Part 2: Implementing SSO Login and Registration
This tutorial will build upon the previous authentication setup to integrate Single Sign-On (SSO) login and registration using Google Oauth. This will allow users to easily sign in using their existing social media accounts, simplifying the signup process and enhancing user experience.
Setting up Google OAuth
First, you must create a project in the
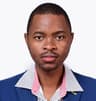
Tech Wizard
Published on β’ π4 min
20 JavaScript Tricks Every Developer Must Know π
JavaScript is a powerful, flexible language, and knowing a few cool tricks can make your code cleaner, faster, and more efficient. JavaScript is the number one programming language in the world, the language of the web, of mobile hybrid apps (likeΒ React Native), of the server s
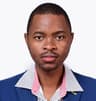
Tech Wizard
Published on β’ π2 min
Free Resources For Web Developers
The web is full of fantastic, free tools that can supercharge your development journey. Whether you need learning platforms, hosting, or design assets, here's a comprehensive guide to help you build, design, and deploy better projects.
πΒ

the don
Published on β’ π4 min
πThe Ultimate Git Cheat Sheetπ
Git is an essential tool for developers, enabling smooth version control, collaboration, and code management across projects. This cheat sheet breaks down the basics of Git, from setup to advanced commands, so you can navigate Gitβs functionality with ease.
1. Setting Up

Tech Tales Team
Published on β’ π3 min
Markdown Tutorial: From Basics to Advanced ππ
Markdown is a lightweight markup language that formats text with simple syntax. It's widely used for blog posts, documentation, and code comments. In this comprehensive guide, we'll cover all the essential Markdown elements, empowering you to easily write beautifully formatted content.
Headings
Headings are used to structure your content and create a hierarchy. Use the pound sig

Tech Tales Team
Published on β’ π3 min
Best Practices on Variable Naming in Javascript
In the realm of JavaScript, crafting clean, readable, and maintainable code is paramount. One fundamental aspect that significantly contributes to code quality is effective variable naming. This blog delves into 12 best practices for variable naming in JavaScript, ensuring your code is not only functional but also comprehensible and maintainable.
1. Embrace let and const: The Modern App

Tech Tales Team
Published on β’ π6 min
How to Write Clean Code β Ten Tips and Best Practices
Clean code is a joy to work with. It is easy to understand, maintain, and extend. In this blog post, we'll explore some tips and best practices for writing clean code, using JavaScript as our example language.
Clean code is a term used to describe computer code that is easy to read, understand, and maintain. Clean code is written in a way that makes it s
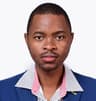
Tech Wizard
Published on β’ π7 min
8 Tools You Need to Build Your First SaaS and Scale Faster
Building a successful SaaS product requires more than just a great idea. You need the right tools to streamline development, manage infrastructure, and optimize your user experience. Luckily enough, you do not need to reinvent the wheel since there are great SaaS products that you can integrate into your project and therefore build and ship faster. This post will guide you through eight essenti
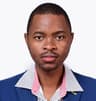
Tech Wizard
Published on β’ π4 min
A Guide to Understanding Web Workers
If you are a web developer who spends a lot of time working, you might think of yourself as a web worker. However, we are sorry this username is taken. The World Wide Web hides many mysteries, and understanding fully how browsers work is challenging. This blog unravels the mystery of web workers, the hidden unpaid interns in websites.
We will explore what web workers are and how they hel