Writing semantic CSS is often hated by developers as it can lead to a lot of bugs and zombie code that unnecessarily bloats the code base. Maintaining a large website with custom CSS is also overwhelming. Luckily, there are CSS frameworks that also come with pre-styled components and thus make web dev faster.
CSS frameworks are pre-prepared libraries that are meant to be used to speed up the process of building websites or web applications. CSS frameworks are used to create familiar and consistent user interfaces, simplify responsive design, and enhance collaboration among development teams.
CSS frameworks come in three different categories: components-based
, utility-first
, and css-in-js
frameworks. Components-based frameworks offer a set of pre-built UI components that developers can plug into their applications to assemble interfaces quickly. The goal is to provide a modular and reusable design system that can help you create consistent and visually appealing web apps without starting from scratch every time.
Utility-first libraries focus on functionality and thus offer CSS classes that can be used in the application to style components. Unline components-based frameworks, these libraries do not offer any component, but classes can be reused.
Lastly, the css-in-js frameworks utilize the dynamic nature of JavaScript to provide a way of writing interactive CSS styles that are performant and based on user data and interactions.
5 Best CSS Frameworks to Use
1. Bootstrap
Bootstrap is a popular component-based framework originally developed by Twitter(now X) and open-sourced in 2011. Bootstrap is one of the most widely used CSS frameworks, with a focus on responsive, mobile-first web design.
Bootstrap offers a robust collection of CSS and JavaScript components, such as its grid system and responsive UI components like buttons, navigation menus, and forms, that streamline the process of building clean and consistent web layouts.
It also has a large community making it easy to find documentation and help. However, Bootstrap can lead to websites that look similar to each other since it is so widely used and its large size can lead to slow loading time. Another consideration is that Bootstrap's default styles may require customization to fit with the design aesthetic of a particular project.
How to Use Bootstrap
To get started, you can add the bootstrap CDN in the header of your HTML, or install the package if you are using single-page applications such as React.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH"
crossorigin="anonymous" />
<title>Bootstrap tutorial</title>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/js/bootstrap.bundle.min.js"
integrity="sha384-YvpcrYf0tY3lHB60NNkmXc5s9fDVZLESaAA55NDzOxhy9GkcIdslK1eN7N6jIeHz"
crossorigin="anonymous"></script>
</head>
<body>
<ul
class="nav nav-pills nav-fill gap-2 p-1 small bg-primary rounded-5 shadow-sm"
id="pillNav2"
role="tablist"
style="
--bs-nav-link-color: var(--bs-white);
--bs-nav-pills-link-active-color: var(--bs-primary);
--bs-nav-pills-link-active-bg: var(--bs-white);
">
<li class="nav-item" role="presentation">
<button
class="nav-link active rounded-5"
id="home-tab2"
data-bs-toggle="tab"
type="button"
role="tab"
aria-selected="true">
Home
</button>
</li>
<li class="nav-item" role="presentation">
<button
class="nav-link rounded-5"
id="profile-tab2"
data-bs-toggle="tab"
type="button"
role="tab"
aria-selected="false">
Profile
</button>
</li>
<li class="nav-item" role="presentation">
<button
class="nav-link rounded-5"
id="contact-tab2"
data-bs-toggle="tab"
type="button"
role="tab"
aria-selected="false">
Contact
</button>
</li>
</ul>
</body>
</html>
You can also install the bootstrap npm package or gem and import only the needed code by running npm install bootstrap @latest
or gem install bootstrap -v 5.3.3
. This helps minimize the code and thus increase loading time.
//src/index.js
import 'bootstrap/dist/css/bootstrap.min.css';
import React from 'react';
function App() {
return (
<div className="container">
<h1>Hello, Bootstrap in React!</h1>
<button className="btn btn-primary">Click Me</button>
</div>
);
}
export default App;
2. Tailwind CSS
Tailwind CSS is by far my best CSS framework and could be at the top of the list, but then I would be biased. Tailwind CSS is a popular utility-first CSS framework that provides a set of pre-defined classes that can be used to style HTML elements.
Unlike Bootstrap, Tailwind is not opinionated and thus gives developers a lot of flexibility. Tailwind CSS is highly customizable and allows you to configure and modify various aspects of the framework to suit your specific needs. It also scales well as unused classes are purged during build time.
However, Tailwind CSS can also cause your code to look like blasphemy. Since Tailwind CSS relies on pre-defined classes, it can result in more HTML markup than is necessary, which may make the HTML code more complex and harder to maintain.
Furthermore, without careful consideration, it's easy to overuse Tailwind CSS classes, resulting in bloated CSS and HTML files, which can negatively impact website performance.
How to Use Tailwind CSS
You can use Tailwind CSS through a CDN by adding the CDN link in the head of your document or by installing it using npm i tailwindcss
and npx tailwindcss init
. Tailwind also offers components but they are in paid plans, although other popular libraries such as daisy UI , FlowBite, Preline
and Shad CN
provide free Tailwind-styled components.
Here is a sample code using the CDN link:
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body>
<h1 class="text-3xl font-bold underline">
Hello world!
</h1>
</body>
</html>
3. Material UI
Material UI is a component-based library for building React ⚛️Applications built and maintained by Google. Material UI offers a modern and visually appealing design system. It features a suite of customization options that make it easy for developers to implement custom design systems on top of the library, making it a popular choice for creating consistent UIs in React applications.
However, Material UI suffers the same fate as Bootstrap in that it is opinionated and websites styled with Material UI tend to look the same. Using pre-styled components can limit your design choices, and Material UI can also increase loading time.
How to Use Material UI
To use Material UI, you need to install the npm package for one of the core UI libraries, with a choice between material UI
, Joy UI
, Base UI
, and MUI system
. The package can be installed by running the npm command:
npm install @mui/material @emotion/react @emotion/styled
You can then import and use components that you need and let the library worry about styling them.
import Button from "@mui/material/Button";
export default function Button() {
return (
<Button variant="contained">Hello World</Button>
);
}
4. Foundation
Foundation is a css-in-js library that brags about being "the most advanced responsive front-end framework in the world". It includes a wide range of pre-designed components and a responsive grid system. It also includes JavaScript plugins for added functionality.
It includes common UI components like Bootstrap but is more utility-focused and gives developers more options for customizing components. With almost too many features, it can be considerably more complex and harder to fully understand how everything works compared to other frameworks.
The framework is less popular and its documentation is less comprehensive compared to other frameworks. Furthermore, Foundation has a smaller user community compared to Bootstrap, so finding support and resources can be more challenging. Some of its features can be less intuitive to use than Bootstrap's.
How to Use Foundation UI
You can install Foundation in your project with a package manager.
npm install foundation-sites
Now you can use its styles and components in your application. Note that you do not import anything but rather utilize its pre-styled classes.
<div class="card" style="width: 300px">
<div class="card-divider">This is a header</div>
<img src="assets/img/generic/rectangle-1.jpg" />
<div class="card-section">
<h4>This is a card.</h4>
<p>It has an easy to override visual style.</p>
</div>
</div>
Since you will probably never use this library, here is a Codepen of a sample of how the library-styled components look like:
5. Mantine UI
I am not sure whether this library makes the list since it is fairly unpopular. Mantine UI is a new CSS framework that includes more than 100 customizable components and 50 hooks to cover you in any situation. The library has over 360,295
weekly downloads on npm.
Unlike other libraries, Mantine comes with everything in one package, including state management utils, tables with pagination, forms with verification, a rich text editor, spotlight, and DOM utils. Mantine also allows you to install only the library that you are using, with an example of @mantine/core
, @mantine/hooks
, @mantine/utils
, @mantine/styles
, @mantine/prism
, and @mantine/datatable
among others.
However, the library is fairly new and thus lacks community support although it is expected to reach a wider audience. If you are working on side projects or your personal projects or if you want to give a life to your idea quickly then you can try this.
How to Use Mantine UI
You can use Mantine UI by installing parts of the library that you need using your package manager.
npm install @mantine/core @mantine/hooks @mantine/form
The library supports various React Frameworks such as vite, gatsby, NextJs, and Remix, and provides templates that are ready to use. After installing the framework, you need to import the CSS file into your root file.
//app.jsx
// core styles are required for all packages
import '@mantine/core/styles.css';
// other css files are required only if
// you are using components from the corresponding package
// import '@mantine/dates/styles.css';
// import '@mantine/dropzone/styles.css';
// import '@mantine/code-highlight/styles.css';
After this, you need to wrap your page with a Mantime provider to get access to all Mantine functionalities such as themes, although this is not necessary. Alternatively, you can directly import the components that you need and use them in your app.
import { createTheme, MantineProvider } from '@mantine/core';
const theme = createTheme({
/** Put your mantine theme override here */
});
function Demo() {
return (
<MantineProvider theme={theme}>
{/* Your app here */}
</MantineProvider>
);
}
//Your App component
import { PinInput } from '@mantine/core';
function OneTimeCodeInput() {
return <PinInput oneTimeCode />;
}
Conclusion
In this blog, we have explored some of the 5 most popular and use CSS libraries/frameworks in 2024. These Libraries can be time-saving as they allow you to quickly bootstrap applications. When choosing the right framework, you need to consider customizability, learning curve, community support, and how the library fits into your project.
Don't choose a framework because it makes your work easier, rather because it fits well with your project!
Tech Wizard
Published on
Tailwind looks like blasphemy
Tutor Juliet
Published on
Tailwind for the win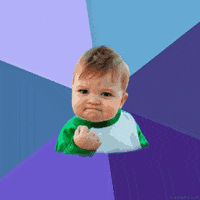
Tech Wizard
• Nov 1, 2024Tech Wizard
• Nov 3, 2024Test response ....